Your cart is currently empty!
A Dynamo Data Migration Tool
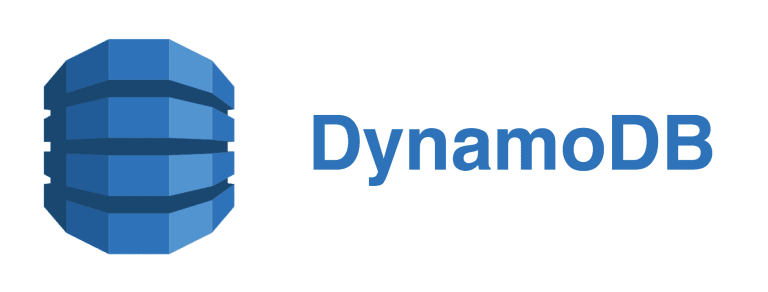
Have you ever wanted to migrate data from one Dynamo DB table to another? I haven’t seen an AWS tool to do this so I wrote one using Python.
import sys
import boto3
## USAGE ############################################################################
## python3 dynamo.py <Source_Table> <destination table> ##
## Requires two profiles to be set in your AWS Config file "source", "destination" ##
#####################################################################################
def dynamo_bulk_reader():
session = boto3.session.Session(profile_name='source')
dynamodb = session.resource('dynamodb', region_name="us-west-2")
table = dynamodb.Table(sys.argv[1])
print("Exporting items from: " + str(sys.argv[1]))
response = table.scan()
data = response['Items']
while 'LastEvaluatedKey' in response:
response = table.scan(ExclusiveStartKey=response['LastEvaluatedKey'])
data.extend(response['Items'])
print("Finished exporting: " + str(len(data)) + " items.")
return data
def dynamo_bulk_writer():
session = boto3.session.Session(profile_name='destination')
dynamodb = session.resource('dynamodb', region_name='us-west-2')
table = dynamodb.Table(sys.argv[2])
print("Importing items into: " + str(sys.argv[2]))
for table_item in dynamo_bulk_reader():
with table.batch_writer() as batch:
response = batch.put_item(
Item=table_item
)
print("Finished importing items...")
if __name__ == '__main__':
print("Starting Dynamo Migrater...")
dynamo_bulk_writer()
print("Exiting Dynamo Migrator")
The process is pretty simple. First, we get all of our data from our source table. We store this in a list. Next, we iterate over that list and write it to our destination table using the ‘Batch Writer’.
The program has been tested against tables containing over 300 items. Feel free to use it for your environments! If you do use it, please share it with your friends and link back to this article!
Leave a Reply